I frequently find myself adding system properties in order to meet ServiceNow’s best practice recommendation on hard-coding sys_ids in code. The best practice states:
Avoid using hard-coded values in scripts, as they can lead to unpredictable results and can be difficult to track down later. Hard coding sys_ids is not recommended, as they may not be the same between instances. Instead, try looking up a value by reference or by creating a property and retrieving the value with gs.getProperty().
https://developer.servicenow.com/dev.do#!/guides/sandiego/now-platform/tpb-guide/scripting_technical_best_practices#do-not-use-hard-coded-values
It very much makes sense why you would not want a sys_id hard-coded in a script (even though sys_ids don’t change in the vast majority of cases…). ServiceNow even recommends using the sys_properties method to get around hard-coding the info in a script (maybe that’s where I learned that trick?).
That’s great for server side scripts, but what about client side? A parallel idea would be to use the Messages table (sys_ui_message), but that’s only useful if you’re writing a client script.
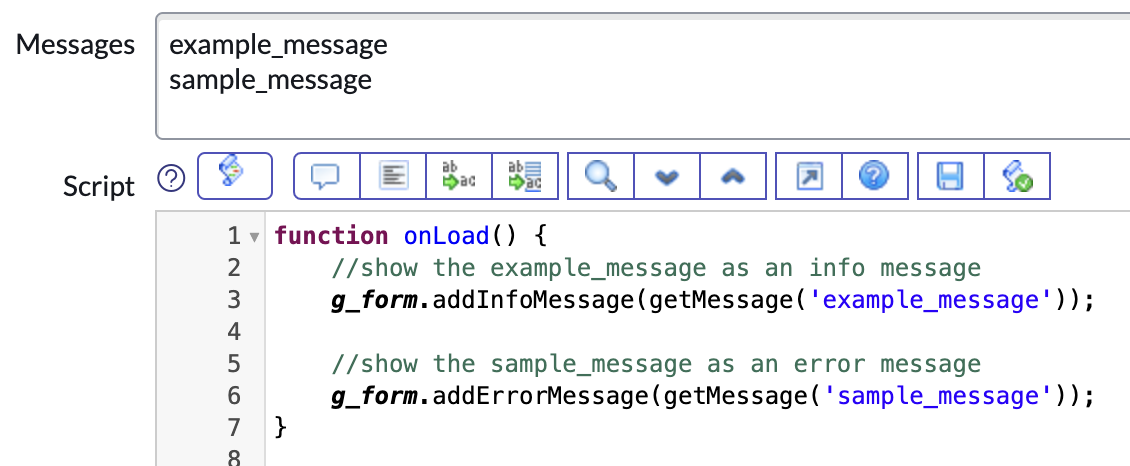
To do this, create a message record in the System UI > Messages table, fill in the Messages field on a client script, and then pull that message into a client script using ‘getMessage(“message_name“)’. (The Messages field might not be on the client script form in your instance, so add it, if needed.)
I don’t think that’s a good solution for two reasons:
- Messages are intended for use in translations and reusable strings throughout the platform
- It doesn’t work in other client-side scripts (there isn’t a Messages field on UI Policy, for example)
So, what’s a dev to do? I decided to solve this by writing a simple GlideAjax function that returns the value of the system property:
var CatalogUtilAjax = Class.create();
CatalogUtilAjax.prototype = Object.extendsObject(AbstractAjaxProcessor, {
getProperty: function() {
var prop = this.getParameter('sysparm_property');
return gs.getProperty(prop);
},
type: 'CatalogUtilAjax'
});
This really assumes that the property exists, so make sure it does (or add error handling as you see fit).
This can be called anywhere GlideAjax can be used with a few simple lines:
var p = new GlideAjax('CatalogUtilAjax');
p.addParam('sysparm_name', 'getProperty');
p.addParam('sysparm_property', '[property_name]');
p.getXMLAnswer(getProperty);
function getProperty(answer) {
//answer = the property value – do with it what you want!
//for example, if the property's value is true, make the "comments" field mandatory:
var isTrue = (answer.toLowerCase() === 'true');
g_form.setMandatory('comments', isTrue);
}
This assumes that there’s an actual value being returned. Be sure it does (or add error handling).
This is a simple solution, but it’s still overly complicated compared to what you would do on the server side. ServiceNow might have their reasons for not providing this as part of the baseline client-side API, but up to now, it just doesn’t exist. Maybe some day, but until then, this solution works just fine!
One thought on “Getting System Properties via GlideAjax”